Developing custom plugins for the Microsoft's Dev Proxy
This post is over a year old, some of this information may be out of date.
For a training project I was working on, I needed to be able to intercept some API calls for some audit logging. To do this, I decided to use Microsoft’s Dev Proxy tool, which you use to simulate, mock, and test APIs.
As the Dev Proxy did not have the functionality I needed out of the box, I decided to develop a custom plugin with the help of Waldek Mastykarz.
In this blog post, I will show you how you can develop custom plugins for the Dev Proxy.
Things you need to get started
Before you can start developing custom plugins for the Dev Proxy, you need to have the following tools installed:
- .NET Core SDK
- Visual Studio Code
- C# Dev Kit Extension
- The Dev Proxy Abstractions DLL
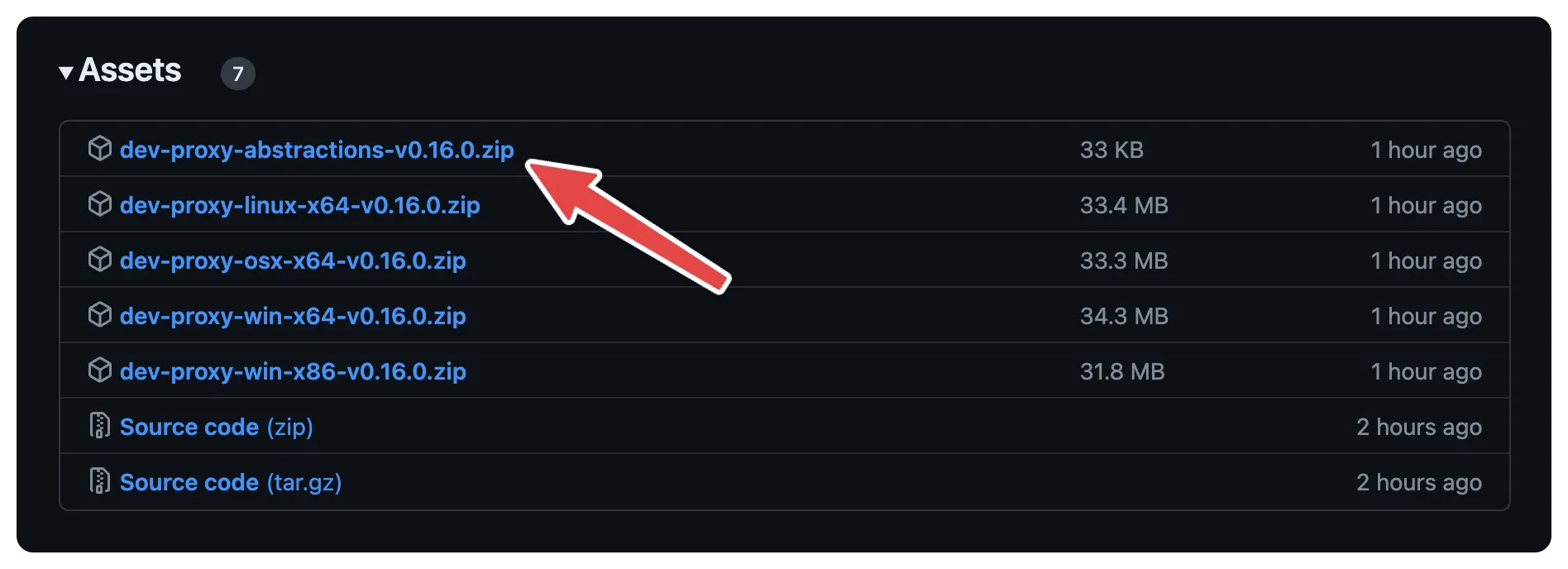
Setting up the project
Once you install all the prerequisites, you can start setting up the project.
- Start creating the new project by running the following command in your terminal:
dotnet new classlib -n DevProxyCustomPlugin
- Next, navigate to the newly created project folder:
cd DevProxyCustomPlugin
- Open the project in Visual Studio Code
- Add the Dev Proxy Abstractions DLL to the project
- Open the
DevProxyCustomPlugin.csproj
file and add the following lines in the project group:
<ItemGroup> <Reference Include="dev-proxy-abstractions"> <HintPath>.\dev-proxy-abstractions.dll</HintPath> <Private>false</Private> <ExcludeAssets>runtime</ExcludeAssets> </Reference></ItemGroup>
- Add the required packages:
dotnet add package Microsoft.Extensions.Configurationdotnet add package Microsoft.Extensions.Configuration.Binderdotnet add package Titanium.Web.Proxy
- Similar to the
dev-proxy-abstractions.dll
configuration, make sure to exclude the new packages’ assets by adding theExcludeAssets
property to thePackageReference
element in theDevProxyCustomPlugin.csproj
file:
<PackageReference Include="Microsoft.Extensions.Configuration" Version="6.0.0"> <ExcludeAssets>runtime</ExcludeAssets></PackageReference>
- Update the
Class1.cs
file with the name of your plugin class:
using Microsoft.DevProxy.Abstractions;using Microsoft.Extensions.Configuration;
namespace DevProxyCustomPlugin;
public class RedirectCalls : BaseProxyPlugin{ public override string Name => nameof(RedirectCalls);
public override void Register(IPluginEvents pluginEvents, IProxyContext context, ISet<UrlToWatch> urlsToWatch, IConfigurationSection? configSection = null) { base.Register(pluginEvents, context, urlsToWatch, configSection); }}
With this in place, you can start developing your custom plugin for the Dev Proxy.
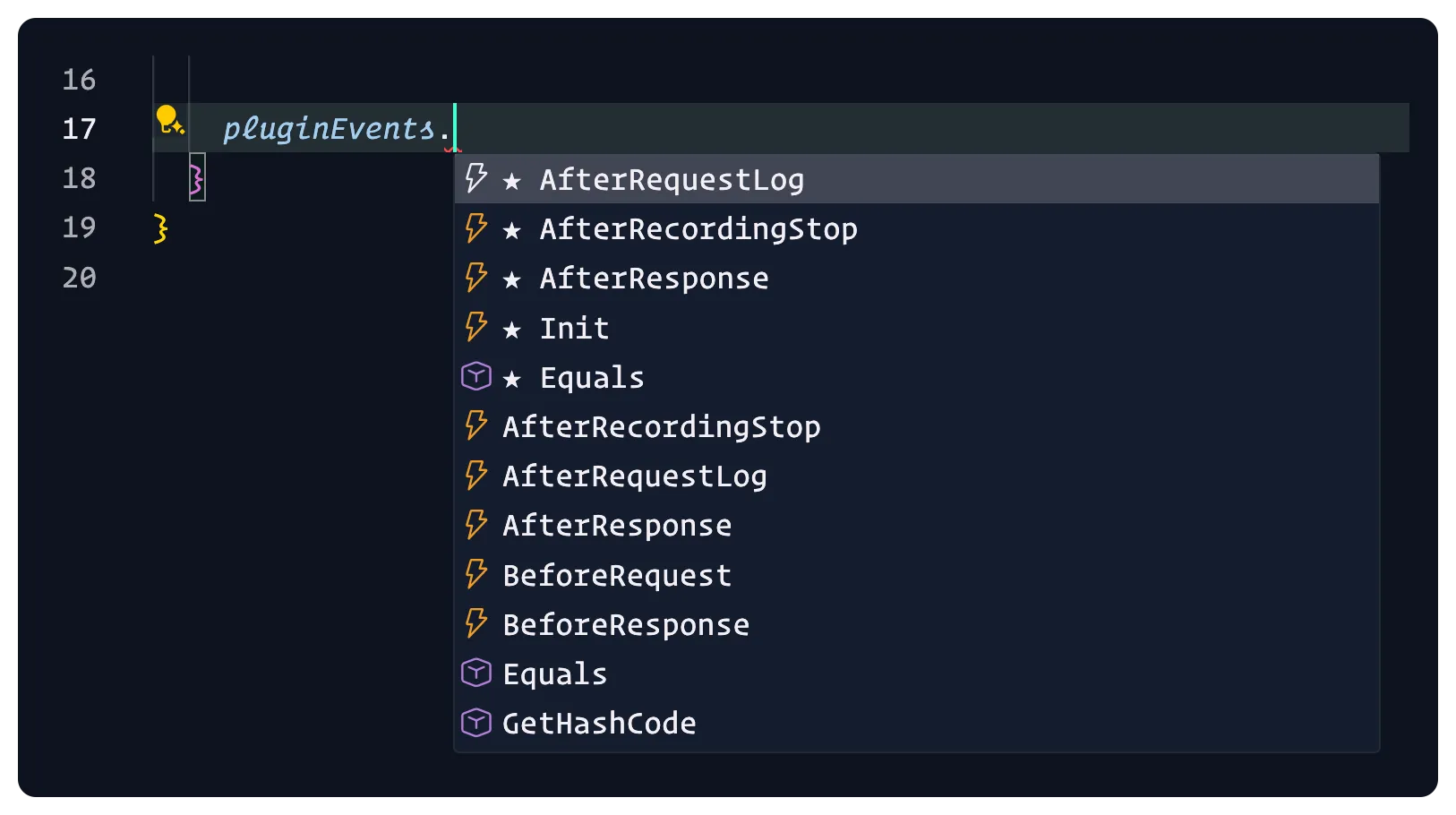
Adding a before-request event handler
You can add a before-request event handler to the plugin to intercept the API calls. In the Register
method, you can add the following code:
public override void Register(IPluginEvents pluginEvents, IProxyContext context, ISet<UrlToWatch> urlsToWatch, IConfigurationSection? configSection = null){ base.Register(pluginEvents, context, urlsToWatch, configSection);
pluginEvents.BeforeRequest += OnBeforeRequest;}
Create a new method, OnBeforeRequest
to handle the event:
private void OnBeforeRequest(object sender, BeforeRequestEventArgs e){ if (_urlsToWatch is null || !e.HasRequestUrlMatch(_urlsToWatch)) { // No match for the URL, so we don't need to do anything return Task.CompletedTask; }
// Replace the following lines with your custom logic if (e.Session.HttpClient.Request.RequestUri.AbsoluteUri.Contains("https://frontmatter.codes")) { var url = e.Session.HttpClient.Request.RequestUri.AbsoluteUri; e.Session.HttpClient.Request.RequestUri = new Uri(url.Replace("https://frontmatter.codes", "http://localhost:3000")); }
return Task.CompletedTask;}
In this example, the OnBeforeRequest
method checks if the request URL contains https://frontmatter.codes
. If it does, it replaces the URL with http://localhost:3000
. That way, I can test the API calls locally while developing the project.
Once you have added the OnBeforeRequest
method’s code, you can build the plugin by running the following command in the terminal:
dotnet build
Testing the plugin
To test the plugin, add it to the Dev Proxy configuration. You can do this by adding the following lines to the devproxyrc.json
file:
{ "$schema": "https://raw.githubusercontent.com/microsoft/dev-proxy/main/schemas/v0.16.0/rc.schema.json", "plugins": [{ "name": "RedirectCalls", "enabled": true, "pluginPath": "./bin/Debug/net8.0/DevProxyCustomPlugin.dll" }], "githubCopilotListener": { "logPath": "./logs" }, "urlsToWatch": [ "https://frontmatter.codes/api/*" ], "labelMode": "text", "logLevel": "debug", "newVersionNotification": "stable"}
Start the Dev Proxy by running the following command in the terminal:
devproxy
Now, you can test the plugin by requesting the URL specified in the urlsToWatch
configuration. In my example, when I call the https://frontmatter.codes/api/stars
API, the request gets redirected to http://localhost:5000/api/stars
, and I can see the response from my local API.
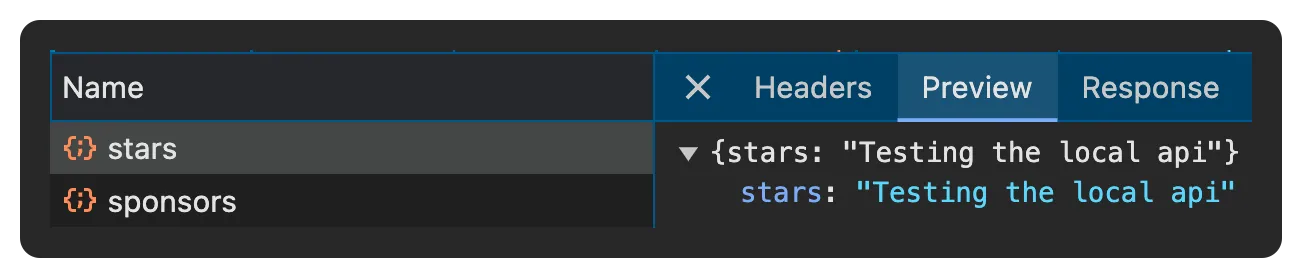
Adding some plugin configuration
To make the plugin more flexible, you can add some configuration options. For instance, you can define the root URL and the local URL to redirect the API calls to.
To do this, you can add the following configuration to the devproxyrc.json
file:
{ "$schema": "https://raw.githubusercontent.com/microsoft/dev-proxy/main/schemas/v0.15.0/rc.schema.json", "plugins": [{ "name": "RedirectCalls", "enabled": true, "pluginPath": "./bin/Debug/net8.0/DevProxyCustomPlugin.dll", "configSection": "redirectCalls" }], "redirectCalls": { "fromUrl": "https://frontmatter.codes", "toUrl": "http://localhost:3000" }, "urlsToWatch": [ "https://frontmatter.codes/api/*" ], "labelMode": "text", "logLevel": "debug", "newVersionNotification": "stable"}
In the RedirectCalls
plugin sections, I added a configSection
property with the value redirectCalls
. This configuration allows me to access the plugin’s configuration.
In the redirectCalls
section, I added the fromUrl
and toUrl
properties, which define the root URL and the local URL to which the API calls should be redirected.
To access the configuration in the plugin, you need to bind the configuration. The whole code for the plugin class should look like this:
using Microsoft.DevProxy.Abstractions;using Microsoft.Extensions.Configuration;
namespace DevProxyCustomPlugin;
public class RedirectCallsConfiguration{ public string? FromUrl { get; set; } public string? ToUrl { get; set; }}
public class RedirectCalls : BaseProxyPlugin{ public override string Name => nameof(RedirectCalls); private readonly RedirectCallsConfiguration _configuration = new();
public override void Register(IPluginEvents pluginEvents, IProxyContext context, ISet<UrlToWatch> urlsToWatch, IConfigurationSection? configSection = null) { base.Register(pluginEvents, context, urlsToWatch, configSection);
configSection?.Bind(_configuration);
pluginEvents.BeforeRequest += OnBeforeRequest; }
private Task OnBeforeRequest(object sender, ProxyRequestArgs e) { if (_urlsToWatch is null || !e.HasRequestUrlMatch(_urlsToWatch)) { // No match for the URL, so we don't need to do anything return Task.CompletedTask; }
var fromUrl = _configuration?.FromUrl ?? string.Empty; var toUrl = _configuration?.ToUrl ?? string.Empty;
if (string.IsNullOrEmpty(fromUrl) || string.IsNullOrEmpty(toUrl)) { return Task.CompletedTask; }
if (e.Session.HttpClient.Request.RequestUri.AbsoluteUri.Contains(fromUrl)) { var url = e.Session.HttpClient.Request.RequestUri.AbsoluteUri; e.Session.HttpClient.Request.RequestUri = new Uri(url.Replace(fromUrl, toUrl)); }
return Task.CompletedTask; }}
With this configuration in place, you can test the plugin with the new configuration options.
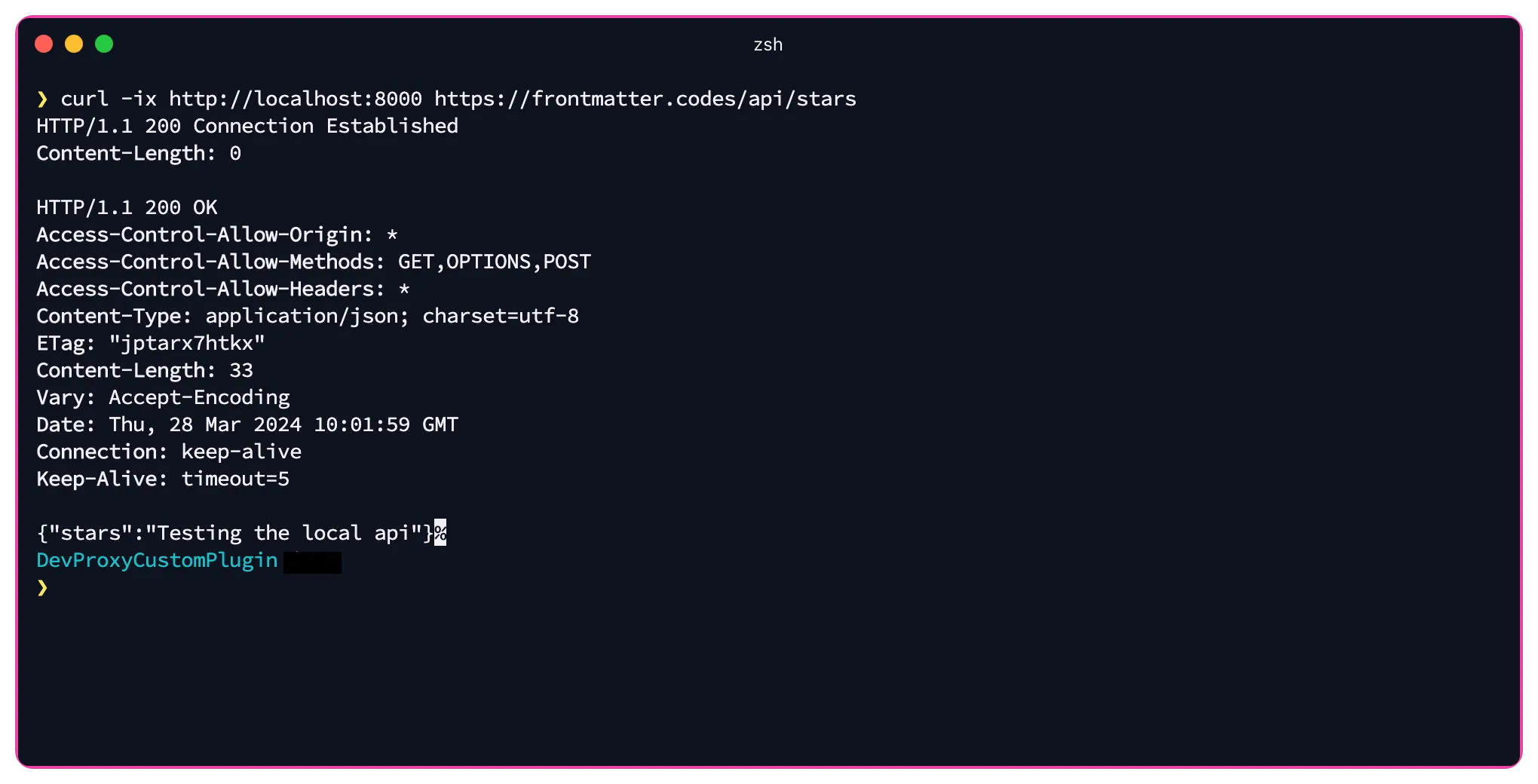
The example above is available on my GitHub devproxy-redirect-plugin repository.
Conclusion
Developing custom plugins for Microsoft’s Dev Proxy is a great way to extend the tool’s functionality. With the help of the Dev Proxy Abstractions DLL, you can easily create custom plugins that can intercept API calls, modify requests and responses, and much more.
Related articles
Using Dev Proxy in your GitHub Actions workflow on macOS
Learn how to use Dev Proxy in a GitHub Actions workflow on macOS hosted VM for intercepting and inspecting your API calls
Using Dev Proxy in your GitHub Actions workflow on Ubuntu
Learn how to use Dev Proxy in a GitHub Actions workflow on an Ubuntu hosted VM for intercepting and inspecting your API calls
Use Playwright with Microsoft Dev Proxy on GitHub Actions
Learn how to use Playwright with Microsoft Dev Proxy on GitHub Actions to easily test your solutions with the same mocked API responses as during development.
Report issues or make changes on GitHub
Found a typo or issue in this article? Visit the GitHub repository to make changes or submit a bug report.
Comments
Let's build together
Manage content in VS Code
Present from VS Code