When developing a custom GitHub Action, you should test and run it locally before pushing it to your repository. Initially, I created a script that allowed me to run it locally, but over the weekend, I found a better way by using the @github/local-action command-line tool.
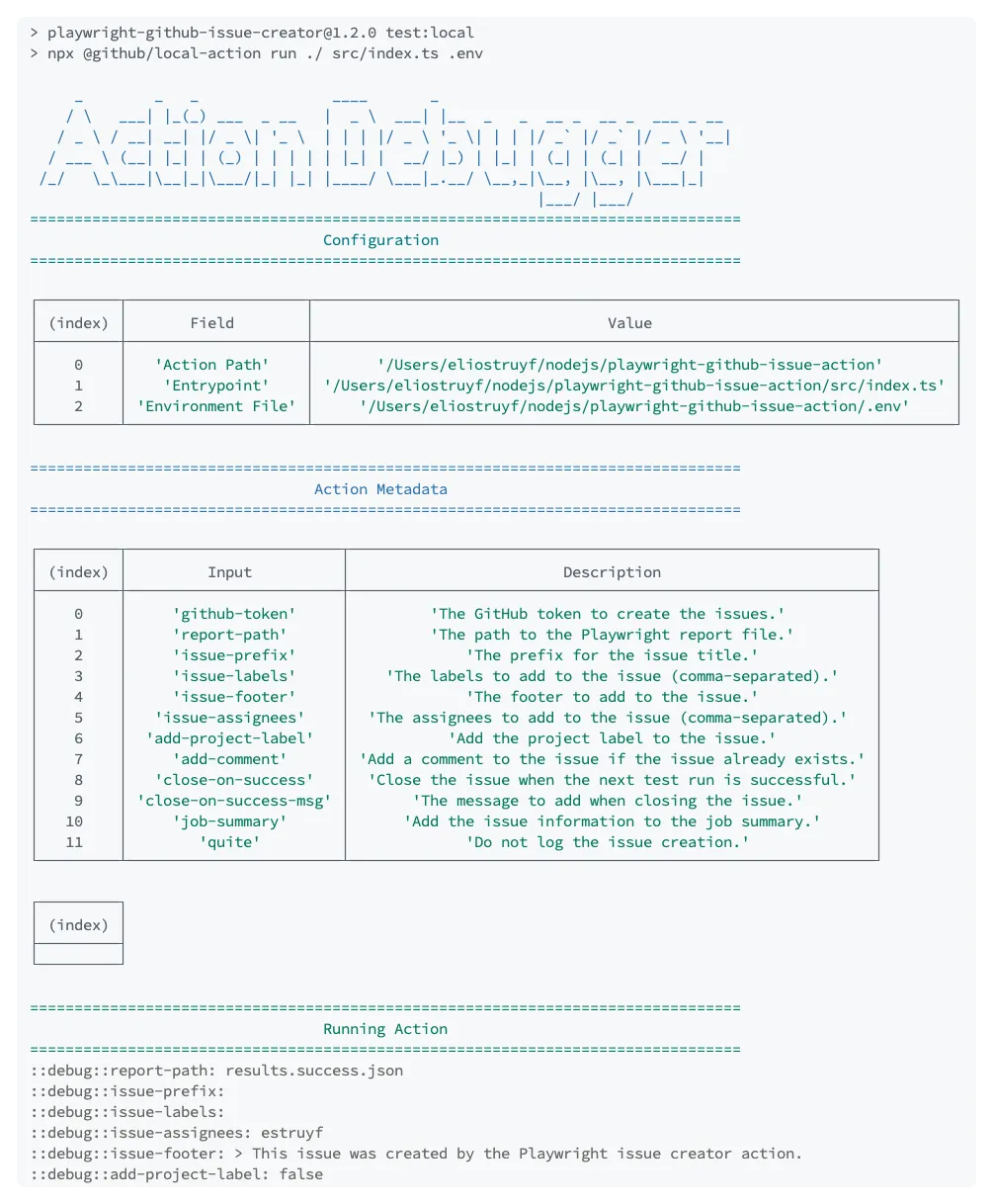
In this post, I will show you how to use the @github/local-action
command-line tool to test your custom GitHub Action locally.
Prerequisites
To start with the @github/local-action
command-line tool, it is best to already have a custom GitHub Action you want to test.
If you don’t have one yet, you can use the JavaScript Action Template or the TypeScript Action Template.
importantThe
@github/local-action
tool only works for JS/TS based GitHub Actions.
Installing the @github/local-action command-line tool
To use the @github/local-action
command-line tool, you need to install it first or use the npx
command to run it without installing it.
|
|
Preparing the environment file
To use the command-line tool, you need to create an environment file to define the input variables and the GitHub variables. Create a new file called .env
in your project and add your configuration.
infoThe name of the environment file is something you can decide, as you need to pass it as an argument to the command-line execution.
infoYou can fine an example configuration of my GitHub Action in the .env.sample file.
Adding your variables
Your GitHub Action might require some input variables to run. You can define these variables in the environment file by adding the INPUT_
prefix to the variable name.
|
|
Defining your GitHub variables
You can also define the GitHub variables in the environment file. These variables define the GitHub context.
In my case, I need to set the GITHUB_REPOSITORY
variable to get the repo
from the @actions/github
its context
object.
|
|
Enabling debugging
You can enable debugging by setting the ACTIONS_STEP_DEBUG
variable to true
.
|
|
Writing the job summary
When your action writes a job summary, you must do the following configuration to enable it locally.
- Create a new file to write the job summary. For example:
summary.md
. - Add the
GITHUB_STEP_SUMMARY
with the path to your summary file.
|
|
infoCheck the file content after an execution of the tool to see your job summary.
Using the @github/local-action command-line tool
Once you have installed the command-line tool and created the .env
file, you can run the following command to test your custom GitHub Action locally.
|
|
The local-action
command takes three arguments:
<path-to-your-action>
: The path to your custom GitHub Action (the folder where youraction.yml
file is located).<path-to-your-entrypoint>
: The path to the entry point of your custom GitHub Action.<path-to-environment-file>
: The path to the environment file you want to use.
All three arguments are required to run the local-action
command.
Here is an example of how I run it for my Playwright Issue Creator GitHub Action:
|
|
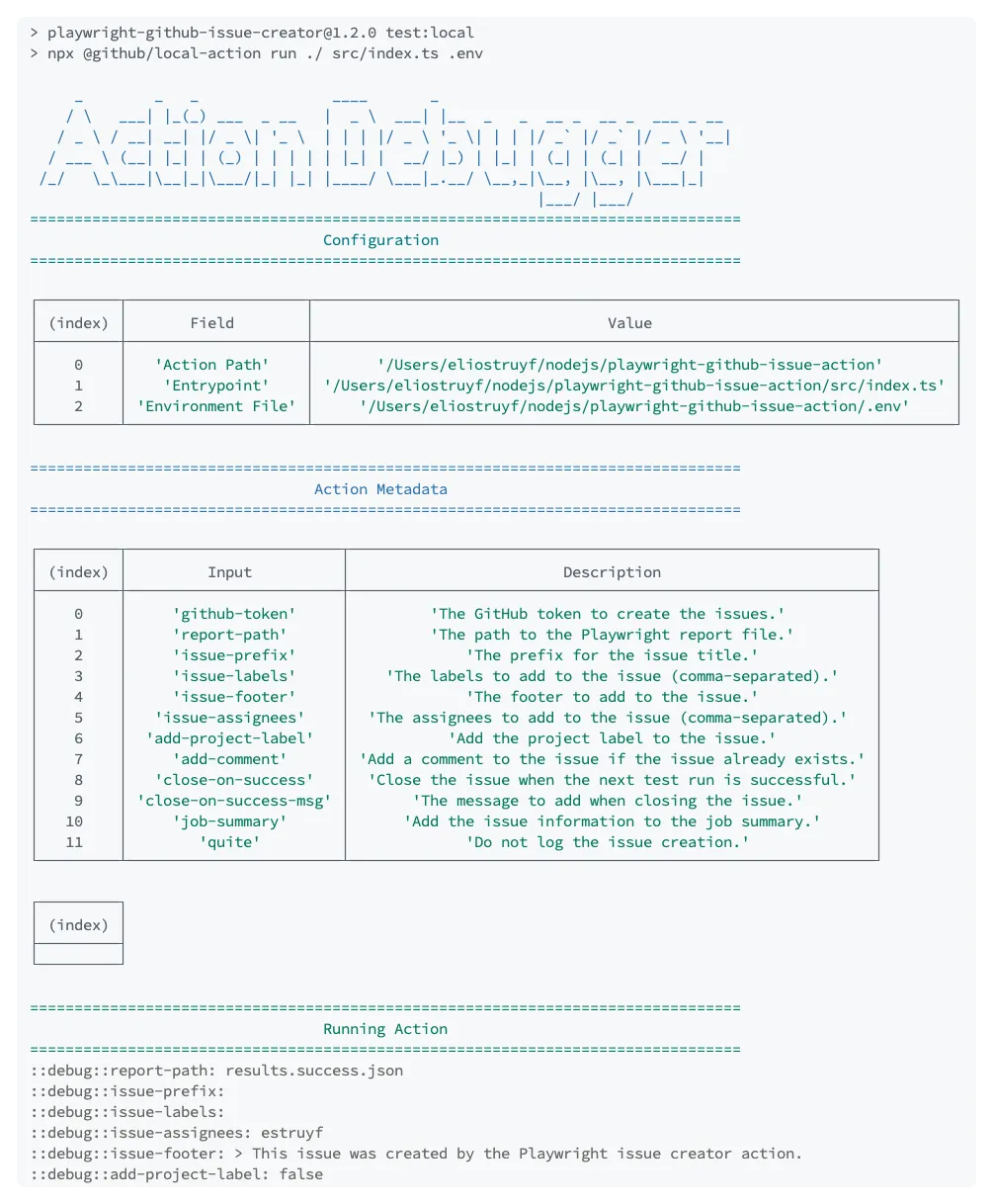
This command-line tool is great during the development process of your custom GitHub Action.
tipYou can also add a Visual Studio Code configuration to allow you to debug your custom GitHub Action directly from your editor. You can check the debugging in VS Code guide for more information.