Integrating Azure Functions into your Astro site
In the previous post, I showed you how to deploy your Astro site to Azure Static Web Apps using the Azure Static Web Apps (SWA) CLI. In this post, I will show you how you can integrate Azure Functions into your Astro site and deploy them using the same SWA CLI.
Why Azure Functions?
Azure Functions are serverless compute services that enable you to run event-triggered code without managing the infrastructure. It allows you to run your code without worrying about the underlying infrastructure. Azure Functions are a great way to run small code that various events can trigger.
The best part is that, in the context of Azure Static Web Apps, you can deploy your Azure Functions alongside your static site. This allows you to build a full-stack application with a static front-end and serverless back-end.
Step 1: Create the Azure Functions API
The first step is to create an Azure Functions API. You can do this using the Azure Functions extension in Visual Studio Code or via the Azure Functions Core Tools.
For this example, I used the Azure Functions Core Tools with the following command:
# Create a new Azure Functions projectfunc init api --worker-runtime typescript --model V4
# Navigate to the new project foldercd api
# Create a new HTTP trigger functionfunc new --template "HTTP trigger" --name http_name
Step 2: Configure the SWA configuration
You must update the file to make your SWA configuration aware of the Azure Functions project. You need to add the following configuration:
{ "$schema": "https://aka.ms/azure/static-web-apps-cli/schema", "configurations": { "astro-func-swa": { "appLocation": "./app", "outputLocation": "dist", "appDevserverUrl": "http://localhost:4321", "apiLocation": "./api", "apiLanguage": "node", "apiVersion": "18", "apiDevserverUrl": "http://localhost:7071" } }}
Step 3: Update the root package.json scripts
Update your package.json
scripts on the root to include the Azure Functions build and dev commands.
{ "scripts": { "build": "npm run build:app && npm run build:api", "build:app": "cd app && npm run build", "build:api": "cd api && npm run build", "dev": "npm-run-all --parallel dev:*", "dev:swa": "swa start", "dev:app": "cd app && npm run dev", "dev:api": "cd api && npm run start", "dev:api_watch": "cd api && npm run watch" }}
Step 4: Run your Astro site and Azure Functions
To run your Astro site and Azure Functions locally, you can use the following command:
npm run dev
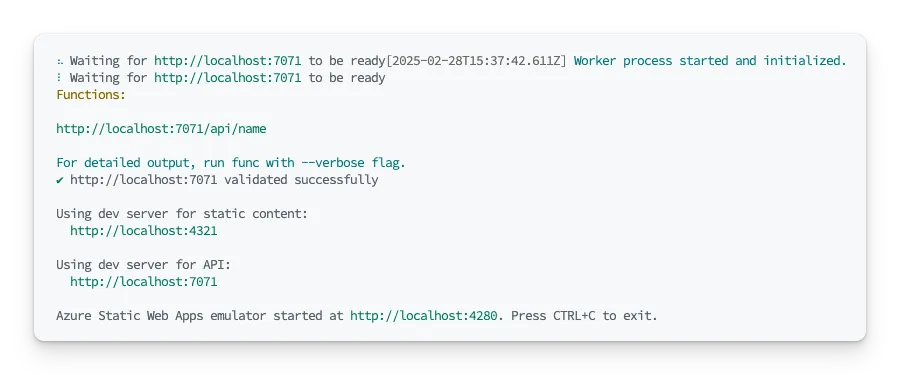
Now, you can test the Azure Functions API on the http://localhost:4280/api/name
endpoint. The nice part is that you don’t need to think about CORS, as both the front and back end are running on the same domain through the Static Web Apps CLI proxy service.
Step 5: Change the code of your Azure Functions
You can now make changes to the Azure Functions, and the development server will automatically pick up on them.
import { app, HttpRequest, HttpResponseInit, InvocationContext,} from "@azure/functions";
export async function http_name( request: HttpRequest, context: InvocationContext): Promise<HttpResponseInit> { return { jsonBody: { firstName: "Elio", lastName: "Struyf", }, };}
app.http("http_name", { methods: ["GET"], authLevel: "anonymous", handler: http_name, route: "name",});
Step 6: Adding the API call to your Astro site
To call the API, I’ll use React to interact with the Azure Functions.
import * as React from "react";
export interface IHelloProps {}
const apiHost = import.meta.env.DEV ? "http://localhost:4280" : "https://<SWA URL>.azurestaticapps.net";
export const Hello: React.FunctionComponent<IHelloProps> = ( props: React.PropsWithChildren<IHelloProps>) => { const [name, setName] = React.useState({ firstName: "", lastName: "" });
const fetchName = async () => { const apiUrl = `${apiHost}/api/name`; try { const response = await fetch(apiUrl); const { firstName, lastName } = await response.json(); return { firstName, lastName }; } catch (error) { console.log(error); console.log(apiUrl); return { firstName: "", lastName: "" }; } };
React.useEffect(() => { const getName = async () => { const fetchedName = await fetchName(); setName(fetchedName); }; getName(); }, []);
if (!name.firstName || !name.lastName) { return <p>Loading...</p>; }
return ( <p> Hello, {name.firstName} {name.lastName} </p> );};
You can now use the Hello
component to display the name fetched from the Azure Functions in the Astro component.
---import { Hello } from "./Hello";---
<Hello client:load />
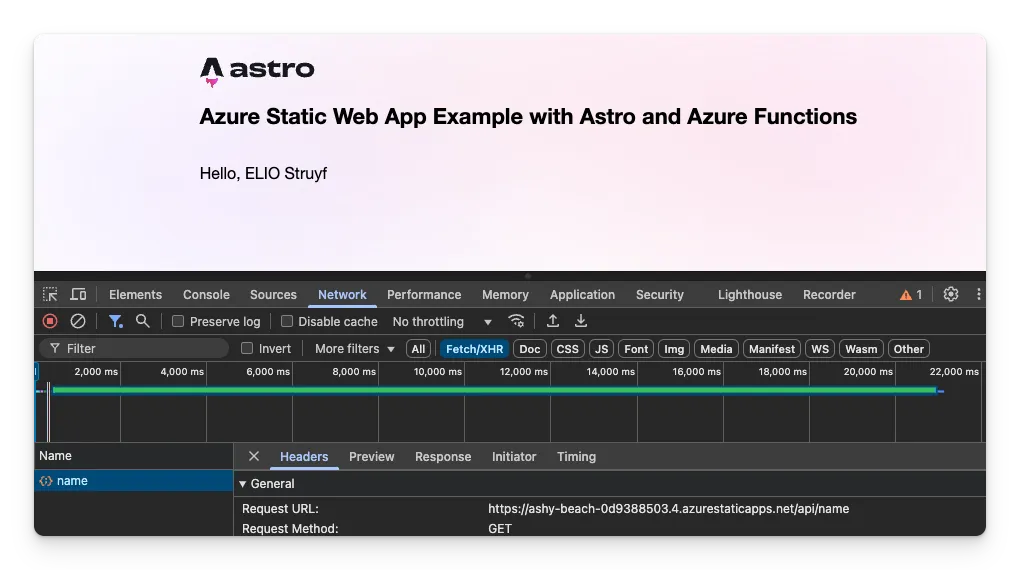
Step 7: Update the GitHub Actions workflow
You need to update the GitHub Actions workflow to ensure that the Azure Functions are deployed with the Astro site.
name: Deployment to Azure Static Web App
on: push: branches: - main
jobs: build_and_deploy_job: runs-on: ubuntu-latest name: Build and Deploy Job steps: - uses: actions/checkout@v4
- name: Setup Node.js uses: actions/setup-node@v4 with: node-version: "18" cache: "npm"
- name: Install dependencies run: | npm ci cd app && npm ci cd .. cd api && npm ci
- name: Build run: npm run build
- name: Clean dependencies run: cd api && npm ci --omit=dev
- name: Deploy run: npx @azure/static-web-apps-cli deploy -d ${{ secrets.AZURE_STATIC_WEB_APPS_API_TOKEN_<ID> }} --env production
Step 8: Deploy your Astro site and Azure Functions
Push your changes to the repository, and GitHub Actions will handle the deployment. Once complete, you can access your site and test the Azure Functions API.
Conclusion
You can add powerful serverless capabilities by integrating Azure Functions with your Astro site. The Static Web Apps CLI simplifies local development by providing a unified proxy, and GitHub Actions automates deployment to production.
Related articles
Fix Azure Function Node.js GitHub Actions Windows workflow
Fixing the Azure Function Node.js GitHub Actions Windows workflow to deploy only production dependencies and exclude the node_modules folder from the artifact.
Deploy Astro to Azure Static Web Apps from GitHub and CLI
Learn to deploy your Astro site to Azure Static Web Apps using GitHub and CLI for a seamless experience.
Which service? Netlify vs Vercel vs Azure Static Web App
Report issues or make changes on GitHub
Found a typo or issue in this article? Visit the GitHub repository to make changes or submit a bug report.
Comments
Let's build together
Manage content in VS Code
Present from VS Code