For a new Visual Studio Code extension called CommitHelper, I wanted to integrate the extension in the Source Control Management (SCM) input. The extension should provide a list of predefined commit messages from which the user can select by using a slash /
in the input field.
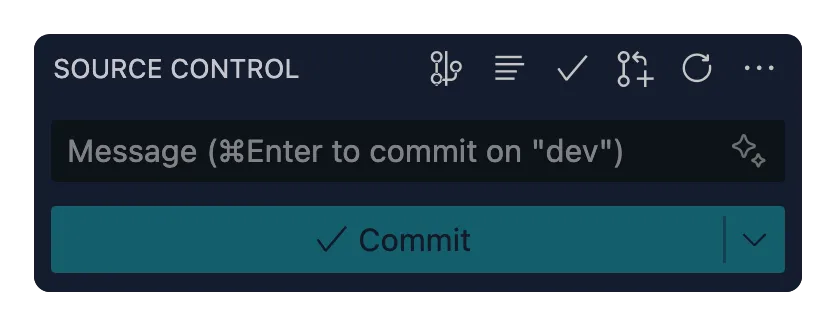
I got the idea from the GitHub Pull Requests extension that provides a similar experience when tagging/linking issues.
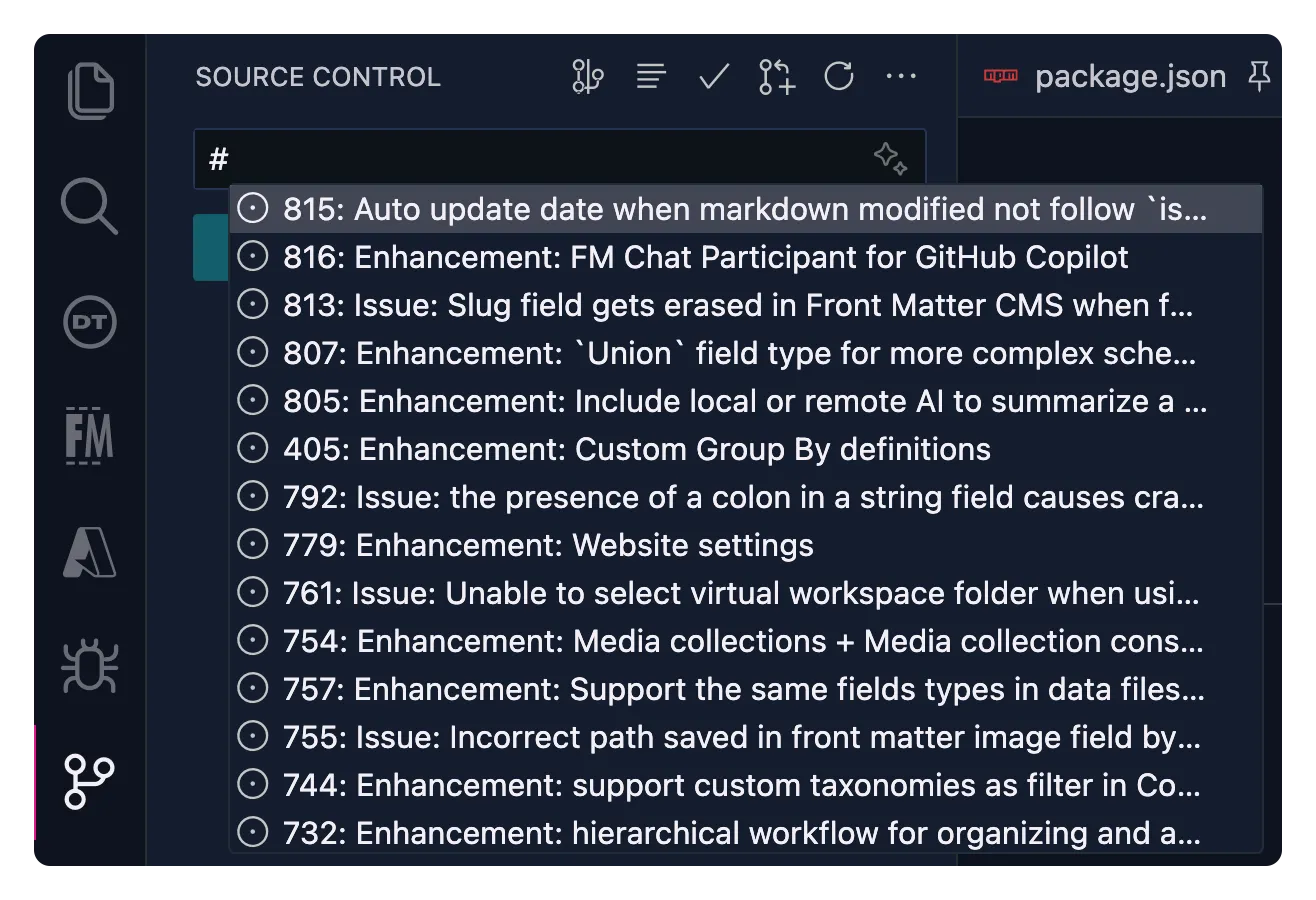
In this article, I will explain how to integrate your Visual Studio Code extension into the SCM input.
Using the VS Code language features
To integrate your extension in the SCM input, you can use the VS Code language features. The language features allow you to provide IntelliSense, hover information, text completion, and more for your language.
The SCM input field also has a language identifier, which you can use to provide text completion for your extension. The language identifier for the SCM input field is scminput
.
In case you want to add text completion to the SCM input field, you can do this by registering a completion item provider for the scminput
language identifier like this:
|
|
The MessageCompletionProvider
class looks like this:
|
|
You can add your completion items in the MessageCompletionProvider class. In the example above, I added two: feat
and fix
. When the user selects one of these items, it inserts the text in the SCM input field.
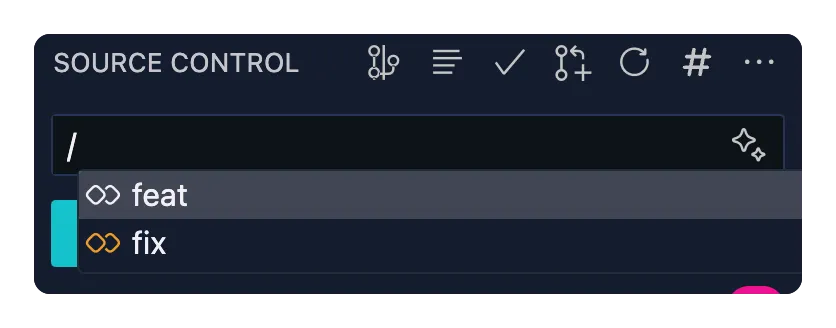
Happy coding!